mirror of
https://github.com/FliegendeWurst/cursive.git
synced 2024-11-10 03:10:41 +00:00
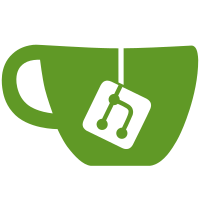
Can be centered, absolute or relative to the previous layer. Can be set independently for each axis.
38 lines
1.4 KiB
Rust
38 lines
1.4 KiB
Rust
extern crate cursive;
|
|
|
|
use cursive::Cursive;
|
|
use cursive::view::{IdView, TextView, Dialog, KeyEventView, Position, Offset};
|
|
|
|
fn show_popup(siv: &mut Cursive) {
|
|
|
|
// Let's center the popup horizontally, but offset it down a few rows
|
|
siv.screen_mut()
|
|
.add_layer_at(Position::new(Offset::Center, Offset::Parent(3)),
|
|
Dialog::new(TextView::new("Tak!"))
|
|
.button("Change", |s| {
|
|
// Look for a view tagged "text". We _know_ it's there, so unwrap it.
|
|
let view = s.find_id::<TextView>("text").unwrap();
|
|
let content: String = view.get_content().chars().rev().collect();
|
|
view.set_content(&content);
|
|
})
|
|
.dismiss_button("Ok"));
|
|
|
|
}
|
|
|
|
fn main() {
|
|
let mut siv = Cursive::new();
|
|
|
|
let content = "Press Q to quit the application.\n\nPress P to open the popup.";
|
|
|
|
siv.add_global_callback('q', |s| s.quit());
|
|
|
|
// Let's wrap the view to give it a recognizable ID, so we can look for it.
|
|
// We add the P callback on the textview only (and not globally),
|
|
// so that we can't call it when the popup is already visible.
|
|
siv.add_layer(KeyEventView::new(IdView::new("text", TextView::new(content)))
|
|
.register('p', |s| show_popup(s)));
|
|
|
|
|
|
siv.run();
|
|
}
|