mirror of
https://github.com/FliegendeWurst/cursive.git
synced 2024-11-10 03:10:41 +00:00
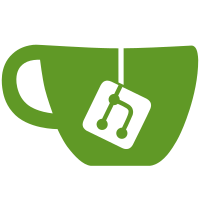
Backends now have to send input to the given `chan::Sender<Event>`. They send these events from a separate thread, allowing selection between input and callbacks. This currently breaks the BearLibTerminal backend, which requires all calls to come from the UI thread. This might not be super-safe for the ncurses backend also. We hope that input and output are separate enough that they can run concurrently without problem.
44 lines
1.2 KiB
Rust
44 lines
1.2 KiB
Rust
extern crate cursive;
|
|
|
|
use cursive::Cursive;
|
|
use cursive::view::Position;
|
|
use cursive::views::LayerPosition;
|
|
use cursive::views::TextView;
|
|
|
|
/// Moves top layer by the specified amount
|
|
fn move_top(c: &mut Cursive, x_in: isize, y_in: isize) {
|
|
// Step 1. Get the current position of the layer.
|
|
let s = c.screen_mut();
|
|
let l = LayerPosition::FromFront(0);
|
|
|
|
// Step 2. add the specifed amount
|
|
let pos = s.offset().saturating_add((x_in, y_in));
|
|
|
|
// convert the new x and y into a position
|
|
let p = Position::absolute(pos);
|
|
|
|
// Step 3. Apply the new position
|
|
s.reposition_layer(l, p);
|
|
}
|
|
|
|
fn main() {
|
|
let mut siv = Cursive::default();
|
|
|
|
// We can quit by pressing `q`
|
|
siv.add_global_callback('q', Cursive::quit);
|
|
// Next Gen FPS Controls.
|
|
siv.add_global_callback('w', |s| move_top(s, 0, -1));
|
|
siv.add_global_callback('a', |s| move_top(s, -1, 0));
|
|
siv.add_global_callback('s', |s| move_top(s, 0, 1));
|
|
siv.add_global_callback('d', |s| move_top(s, 1, 0));
|
|
|
|
// Add window to fly around.
|
|
siv.add_layer(TextView::new(
|
|
"Press w,a,s,d to move the window.\n\
|
|
Press q to quit the application.",
|
|
));
|
|
|
|
// Run the event loop
|
|
siv.run();
|
|
}
|